Abstraction
Are you using any 3rd-party componet or ActiveX, which is not stable enough, in your program? You will get a GPF dialog if the componet in your program goes abnormal. That is not your problem totally, but actually your program crashed. There's a Windows API to prevent showing GPF dialog, you may adapt this solution if the crash can be ignored.
Code Snippet
UINT uPrevErrMode = SetErrorMode(SEM_NOGPFAULTERRORBOX);
Do you not know what GPF is?
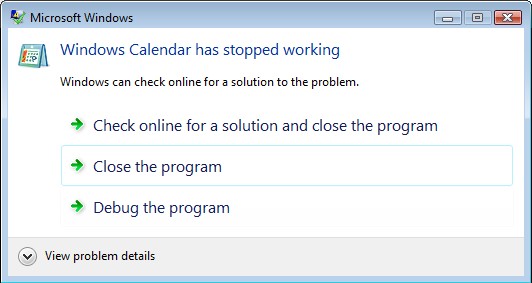
Reference on MSDN